mirror of
https://github.com/casdoor/casdoor.git
synced 2025-07-04 05:10:19 +08:00
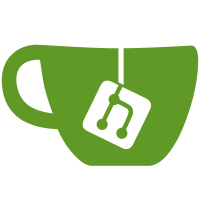
* feat: add sync module to bi-sync mysql * feat: fix the delay problem * feat: fix go mod * feat: fix the varchar(100) parse error * fix: fix go.mod space inconsistency * fix: fix go.mod space inconsistency * fix: use sql builder instead of concatenation * fix: remove serverId * fix: fix file is not `gofumpt`-ed (gofumpt) error * feat: add mysql bi-sync * feat: fix some data inconsistency problems * feat: add function atuo get server uuid * fix: encapsulate the struct to optimize the code * fix: fix incorrect Casdoor version in system info page * fix: fix incorrect root path * Update sysytem_test.go * feat: add aheadCnt means that the commit is ahead of version several times --------- Co-authored-by: hsluoyz <hsluoyz@qq.com>
93 lines
2.3 KiB
Go
93 lines
2.3 KiB
Go
// Copyright 2022 The Casdoor Authors. All Rights Reserved.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package controllers
|
|
|
|
import (
|
|
"github.com/casdoor/casdoor/object"
|
|
"github.com/casdoor/casdoor/util"
|
|
)
|
|
|
|
type SystemInfo struct {
|
|
MemoryUsed uint64 `json:"memory_used"`
|
|
MemoryTotal uint64 `json:"memory_total"`
|
|
CpuUsage []float64 `json:"cpu_usage"`
|
|
}
|
|
|
|
type GitRepoInfo struct {
|
|
AheadCnt int `json:"ahead_cnt"`
|
|
Commit string `json:"commit"`
|
|
Version string `json:"version"`
|
|
}
|
|
|
|
// GetSystemInfo
|
|
// @Title GetSystemInfo
|
|
// @Tag System API
|
|
// @Description get user's system info
|
|
// @Param id query string true "The id ( owner/name ) of the user"
|
|
// @Success 200 {object} object.SystemInfo The Response object
|
|
// @router /get-system-info [get]
|
|
func (c *ApiController) GetSystemInfo() {
|
|
id := c.GetString("id")
|
|
if id == "" {
|
|
id = c.GetSessionUsername()
|
|
}
|
|
|
|
user := object.GetUser(id)
|
|
if user == nil || !user.IsGlobalAdmin {
|
|
c.ResponseError(c.T("auth:Unauthorized operation"))
|
|
return
|
|
}
|
|
|
|
cpuUsage, err := util.GetCpuUsage()
|
|
if err != nil {
|
|
c.ResponseError(err.Error())
|
|
return
|
|
}
|
|
|
|
memoryUsed, memoryTotal, err := util.GetMemoryUsage()
|
|
if err != nil {
|
|
c.ResponseError(err.Error())
|
|
return
|
|
}
|
|
|
|
c.Data["json"] = SystemInfo{
|
|
CpuUsage: cpuUsage,
|
|
MemoryUsed: memoryUsed,
|
|
MemoryTotal: memoryTotal,
|
|
}
|
|
c.ServeJSON()
|
|
}
|
|
|
|
// GitRepoVersion
|
|
// @Title GitRepoVersion
|
|
// @Tag System API
|
|
// @Description get local github repo's latest release version info
|
|
// @Success 200 {string} local latest version hash of casdoor
|
|
// @router /get-release [get]
|
|
func (c *ApiController) GitRepoVersion() {
|
|
aheadCnt, commit, version, err := util.GetGitRepoVersion()
|
|
if err != nil {
|
|
c.ResponseError(err.Error())
|
|
return
|
|
}
|
|
|
|
c.Data["json"] = GitRepoInfo{
|
|
AheadCnt: aheadCnt,
|
|
Commit: commit,
|
|
Version: version,
|
|
}
|
|
c.ServeJSON()
|
|
}
|