mirror of
https://github.com/casdoor/casdoor.git
synced 2025-07-03 12:30:19 +08:00
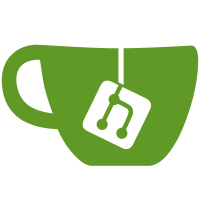
* fix: add response when web file not found The error flow is as follows: Assuming my directory structure is as follows: ```tree ├── GitHub │ ├── casdoor # code repository ├── casdoor # compiled binary file ``` Execute the program in the `GitHub` directory: ```bash ./casdoor/casdoor ``` The working directory at this time is `GitHub`. According to the code: ```go func StaticFilter(ctx *context.Context) { urlPath := ctx.Request.URL.Path /// omitted path := "web/build" if urlPath == "/" { path += "/index.html" } else { path += urlPath } if !util.FileExist(path) { path = "web/build/index.html" } if !util.FileExist(path) { return } /// omitted } ``` If the user accesses `/`, according to this code, the returned value is actually `web/build/index.html`. But the current directory is GitHub, and there is no `web/build/index.html` file. According to the following code, it will directly return: ```go if !util.FileExist(path) { return } ``` Then in `main.go`: ```go beego.InsertFilter("*", beego.BeforeRouter, routers.StaticFilter) beego.InsertFilter("*", beego.BeforeRouter, routers.AutoSigninFilter) beego.InsertFilter("*", beego.BeforeRouter, routers.CorsFilter) beego.InsertFilter("*", beego.BeforeRouter, routers.ApiFilter) beego.InsertFilter("*", beego.BeforeRouter, routers.PrometheusFilter) beego.InsertFilter("*", beego.BeforeRouter, routers.RecordMessage) ``` The introduction of `beego.InsertFilter` is as follows: ``` func InsertFilter(pattern string, pos int, filter FilterFunc, params ...bool) *App InsertFilter adds a FilterFunc with pattern condition and action constant. The pos means action constant including beego.BeforeStatic, beego.BeforeRouter, beego.BeforeExec, beego.AfterExec and beego.FinishRouter. The bool params is for setting the returnOnOutput value (false allows multiple filters to execute) ``` When the `params` parameter is `false`, it runs multiple filters. The default is `true`. So normally, if ```go beego.InsertFilter("*", beego.BeforeRouter, routers.StaticFilter) ``` response something, the following filters will not be executed. But because the file does not exist, the function directly returns, causing the subsequent filters to continue executing. When it reaches ```go beego.InsertFilter("*", beego.BeforeRouter, routers.ApiFilter) ``` it will start to check permissions: ``` subOwner = anonymous, subName = anonymous, method = GET, urlPath = /login, obj.Owner = , obj.Name = , result = deny ``` Then it will report this error: ```json { "status": "error", "msg": "Unauthorized operation", "data": null, "data2": null } ``` The solution should be: ```go func StaticFilter(ctx *context.Context) { urlPath := ctx.Request.URL.Path /// omitted path := "web/build" if urlPath == "/" { path += "/index.html" } else { path += urlPath } if !util.FileExist(path) { // todo: response error: page not found return } /// omitted } ``` * Update static_filter.go --------- Co-authored-by: hsluoyz <hsluoyz@qq.com>